Introduction to Control Structures: Part 1
Posted on Mar 02, 2011 @ 04:04 AM by ThePhantom
Programming languages by default execute in sequence; that is they execute line by line. This is very useful since you want to have an order; but how about if we need to make decisions and evaluate some input and decide which path to take depending on that input. Well, that’s when we use Control Structures.
Control Structures allow us, programmers, to change that default sequential execution. In most Programming Languages such as PHP, C++, C, C#, Java, JavaScript, and others, we have Control Structures.
The first Control Structure we are going to talk about is the “if” and “if … else”. What this structure does is to evaluate the condition of the “if” statement and determine if it’s true or false; then if it’s true executes the statements inside the “if” body, otherwise executes statements in the else body or continues executing the rest of the program.
The syntax is similar in different programming languages; although it might change a bit. The general syntax is as follow:NOTICE that we did not use the curly braces “{}” in any of the “if … else” statements. This is because there is no need to use the braces if there is only one statement in the body of the “if .. else” statement; but it is a good programming practice to always put the braces, even though if there is only one statement inside the body if the “if … else” statements.
NOTE: the else statement must be always associated with an if statement, that is it must be immediately after the if statement.
These condition statements can be nested. That is to put one inside the other one. For instanceThese nested control structures can be rewritten using “else if”. For example, the above code can be rewritten as:Let’s take a look at the following codeIn the previous code both sections will accomplish the same task but with the only difference that Section 1 will do it faster and more efficiently than Section 2. That is because when a condition in Section 1 is evaluated to true then the program doesn’t evaluate the rest of the conditions; whereas in Section 2 when one condition is evaluated to true the program will keep evaluating the remaining conditions.
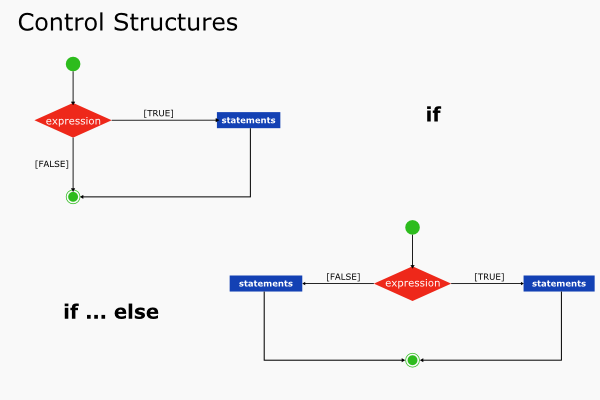
if( condition ) statement if ( condition ) statement else statement
if ( condition ) statement else if ( condition1 ) statement else statement
if( condition ) statement else if ( condition1 ) statement else statement
// Section 1 if( condition ) statement else if ( condition1 ) statement else if ( condition2 ) statement else if ( condition3 ) statement // Section 2 if( condition ) statement if ( condition1 ) statement if ( condition2 ) statement if ( condition3 ) statement
Comments:

